Embedded Software Roadmap : Are you eager to dive into the world of embedded systems but don’t know where to start? This beginner-friendly roadmap will guide you step-by-step, from basic concepts to real-time projects, helping you build a strong foundation and become a successful embedded software engineer in 2025.
Embedded Software Roadmap
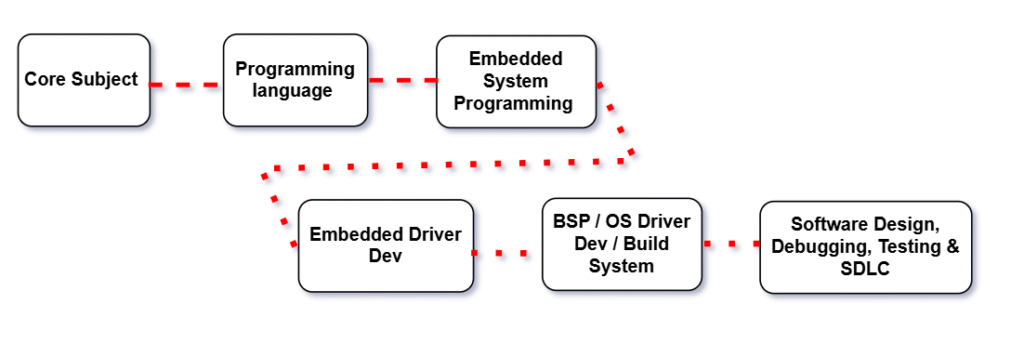
Step 1: Master the Core Subjects of Embedded Systems
1.1 Digital Electronics
Digital Electronics is the foundation of modern computing and embedded systems. It harnesses the power of binary logic—0s and 1s—to design systems that are precise, reliable, and scalable. As the first step in the Embedded Software Roadmap, mastering digital electronics is essential. From microprocessors to embedded controllers, digital electronics enables the creation of intelligent hardware through logic gates, Boolean algebra, and state-driven systems. This knowledge sets the stage for developing efficient and robust embedded software.
At its core, digital electronics replaces analog ambiguity with digital precision, making it the heart of systems like communication devices, automation units, robotics, and consumer electronics. By combining combinational and sequential circuits, engineers can design everything from simple adders to complex programmable logic devices like FPGAs.
With digital design, we build the brains of technology—machines that think, decide, and act based on logic and encoded instructions. It’s not just about electronics—it’s about empowering innovation through logical architecture.
Understand how electronic signals represent data:
- Number systems: Binary, Hexadecimal, BCD
- Logic Gates: AND, OR, NOT, NAND, NOR, XOR
- Flip-Flops and Registers
- Combinational vs Sequential Circuits
- Multiplexers, Encoders, Decoders
Popular Microcontrollers to Explore:
- AVR: ATmega328P (used in Arduino)
- ARM: STM32, ESP32, nRF52
1.2 Operating Systems (OS)
Digital Electronics is the foundation of modern computing and embedded systems. It harnesses the power of binary logic—0s and 1s—to design systems that are precise, reliable, and scalable. As the first step in the Embedded Software Roadmap, mastering digital electronics is essential. From microprocessors to embedded controllers, digital electronics enables the creation of intelligent hardware through logic gates, Boolean algebra, and state-driven systems. This knowledge sets the stage for developing efficient and robust embedded software.
As we move forward in the Embedded Software Roadmap, we encounter the critical role of Operating Systems.
An Operating System (OS) is the unseen force that transforms hardware into a functional and responsive digital environment. Acting as the central coordinator, it manages everything—from running applications and allocating memory to handling user input and controlling hardware components.
It provides an abstraction layer, allowing developers and users to interact with complex machines through simple, intuitive interfaces. Whether it’s scheduling tasks, managing filesystems, or ensuring secure multitasking, the OS silently enforces structure and efficiency beneath every click and tap.
From real-time embedded platforms like FreeRTOS, QNX, and VxWorks to general-purpose systems like Linux, Windows, and macOS, Operating Systems are not just software—they are digital ecosystems that balance performance, reliability, and usability.
Why it’s important: Many embedded systems run on RTOS (like FreeRTOS, VxWorks) or Embedded Linux. Understanding OS concepts helps you manage processes, memory, and scheduling efficiently.
Topics to Cover:
- Process vs Thread
- Memory Management (Heap, Stack, Paging)
- CPU Scheduling Algorithms
- Interprocess Communication (IPC): semaphores, mutex, pipes
- Real-Time Operating Systems (RTOS): concepts of tasks, preemption, priority inversion
- Embedded Linux basics: init system, daemons, file system layout
1.3 Computer Architecture
Computer Architecture defines the conceptual design and operational framework of a computer system. It is the strategic art of organizing hardware components and establishing how they interact to execute instructions efficiently and reliably.
In the Embedded Software Roadmap, understanding computer architecture is a critical step—especially for developers working close to the hardware. This architectural blueprint includes the internal structure of the CPU—such as the control unit, ALU, and registers—as well as memory hierarchy, I/O mechanisms, and instruction sets. It governs how software communicates with hardware, how data moves through the system, and how performance can be improved using techniques like pipelining, caching, and parallel processing.
Why it matters: Embedded development often involves bare-metal programming, writing assembly code, or optimizing C/C++ for resource-constrained environments. A solid grasp of computer architecture empowers developers to write efficient code, debug at the hardware level, and fine-tune performance for real-time applications.
In essence, computer architecture transforms raw silicon into a purposeful computing engine—balancing speed, power, and scalability to meet the demands of modern embedded systems..
Topics to Cover:
- Instruction Set Architecture (ISA): RISC vs CISC
- ARM Cortex-M and ARMv7 architecture
- Registers, ALU, Control Unit
- Pipelining and hazards
- Caches (L1/L2), memory hierarchy
- Memory-mapped I/O
- Endianness
1.4 Computer Networks
As embedded systems evolve, connectivity becomes increasingly vital. Computer Networks form the backbone of modern communication, enabling devices to share data, resources, and services—whether they’re part of a smart home, a vehicle, or a global cloud infrastructure.
In the Embedded Software Roadmap, understanding computer networks is crucial for building connected and intelligent embedded systems. Networks are built on layered protocols, starting from physical connections and data link layers to higher-level transport and application services. These layers work together to define how information is packaged, transmitted, routed, and received reliably and securely.
From wired LANs in industrial automation to wireless technologies powering IoT ecosystems, networks allow embedded devices to interact in real-time, share sensor data, receive firmware updates, and integrate with cloud platforms.
Why it’s important: Many modern embedded systems are no longer standalone. Devices often operate as part of a larger connected environment, making it essential to understand networking protocols like TCP/IP, UDP, MQTT, HTTP, and Bluetooth. This knowledge allows developers to implement secure, scalable, and efficient communication in embedded applications.
More than just infrastructure, computer networks serve as the digital nervous system of embedded and IoT applications—ensuring smooth, synchronized, and intelligent operations across distributed systems.
Topics to Cover:
- OSI Model and TCP/IP
- IP, UDP, TCP – Packet structures, handshakes
- HTTP/HTTPS protocols
- MQTT and CoAP (for IoT)
- Bluetooth, Wi-Fi, ZigBee (basic wireless comm.)
- Socket programming basics
Step 2: Master the Programming Language
At the heart of embedded software development lies programming languages—tools that transform ideas into functional systems. In the Embedded Software Roadmap, mastering the right programming languages is a fundamental step. These languages enable developers to write efficient, optimized code that directly controls hardware and processes data in real-time.
For embedded systems, C and C++ are the most widely used languages due to their efficiency, low-level hardware access, and performance. C is often preferred for bare-metal development because it allows precise control over system resources, while C++ introduces object-oriented features that enhance code structure, maintainability, and scalability.
In addition to C/C++, many embedded developers also explore Python for higher-level tasks like testing, automation, and scripting, as well as Assembly for low-level performance tuning. Understanding these languages provides the flexibility to tackle various embedded development challenges, whether you’re programming microcontrollers, designing device drivers, or developing real-time applications.
Why it’s important: Mastery of these languages allows embedded engineers to write efficient, low-level code that interacts directly with hardware, ensuring fast execution and minimal resource consumption. Additionally, knowledge of object-oriented programming in C++ supports building modular and maintainable systems, which is crucial for complex embedded projects.
In essence, mastering programming languages empowers embedded software engineers to translate hardware requirements into functional code—balancing performance, resource utilization, and reliability in every line of code.
2.1 Start with Embedded C Programming
Why C?
C is the de facto standard for embedded development because of its:
- Low-level access to memory (pointers)
- Speed and minimal overhead
- Hardware-level register manipulation
- Portability across microcontrollers
Topics to Master in C:
Basics:
- Data types (
uint8_t
,uint16_t
, etc. from<stdint.h>
) - Operators, Control flow, Functions
Intermediate:
- Pointers, Arrays, Strings
- Bitwise operations (
|
,&
,^
,~
,<<
,>>
) - Structs, Enums, Typedef
- Header files and Modular programming
- Makefiles and Compilation
Advanced:
- Memory management: Stack vs Heap
const
,volatile
,static
,extern
- Inline functions, Macros, Preprocessor directives
- Interrupt Service Routines (ISRs) in C
- Working with hardware registers (
*(volatile uint32_t*)0x40021000 = 0x01;
)
Step 2.1: C++
Once you’ve got a handle on C, it’s time to go deeper into C++, which is widely used in automotive, RTOS-based, and IoT embedded systems.
a)Learn Basic C++
Keywords: basic C++ tutorial, C++ syntax, C++ for beginners
Start with the fundamentals:
- Data types & variables
- Conditionals and loops
- Functions and recursion
- Arrays and strings
- Pointers and references
- Dynamic memory (
new
,delete
) - Namespaces, inline functions, function overloading
🎯 Practice via platforms like LeetCode, HackerRank, or using mini console-based apps.
b) Understand OOPs Concepts
In the world of embedded software development, Object-Oriented Programming (OOP) is a powerful design paradigm that structures code around real-world entities. Instead of focusing solely on procedures or functions, OOP organizes software into objects—modular, reusable units that encapsulate both data and behavior. This approach makes the code more intuitive, maintainable, and scalable.
In the Embedded Software Roadmap, understanding OOP concepts like encapsulation, inheritance, polymorphism, and abstraction is essential for developing robust embedded systems, especially as applications grow in complexity. By focusing on what things are (objects) and what they do (methods), developers can model real-world systems more naturally, improving both code readability and flexibility.
Why it’s important: For embedded systems, OOP allows you to write cleaner, more organized code. It enables better reuse of software components, which can be critical when developing complex systems with limited resources. By applying OOP principles, embedded developers can design more scalable and maintainable systems, while also reducing errors and simplifying debugging.
In essence, mastering OOP in embedded software development provides the tools to design systems that are not only functional but also easier to manage and extend over time—ensuring long-term efficiency and adaptability.
Object-Oriented Programming is what gives C++ its real power.
- Classes and Objects
- Encapsulation, Abstraction
- Inheritance (single, multiple, hybrid)
- Polymorphism (compile-time & runtime)
- Constructors and Destructors
this
pointer, friend functions, operator overloadingconst
,static
,virtual
, pure virtual functions
Note: In embedded, avoid dynamic memory allocation and deep inheritance trees.
c)Learn STL (Standard Template Library)
The Standard Template Library (STL) is a powerful feature of C++ that brings the elegance of generic programming to everyday development. It offers a rich set of predefined classes and functions—ready to manage data structures and algorithms with optimal efficiency and minimal effort.
STL is useful even in high-end embedded systems or while writing test utilities.
Key STL components to learn:
- Containers:
vector
,list
,deque
,stack
,queue
,set
,map
- Algorithms:
sort
,find
,count
,accumulate
,for_each
- Iterators: Input, Output, Forward, Bidirectional, Random access
- Function Objects & Lambda Expressions
- Templates: Function and class templates
d)Practice DSA (Data Structures & Algorithms)
Data Structures and Algorithms (DSA) form the strategic core of software development, providing the tools and techniques needed to organize data and solve problems efficiently. While data structures define how information is stored, algorithms dictate the logic to process and transform it.
From managing dynamic memory with linked lists and trees to optimizing decisions through sorting, searching, and graph traversal, DSA equips developers to think critically and code smartly. It transforms brute-force solutions into optimized, scalable approaches—essential in everything from system design to real-time applications.
Mastering DSA isn’t just about writing code—it’s about developing a problem-solving mindset that balances time, space, and logic to build high-performance software in the real world.
Embedded engineers may not need complex DSA every day, but knowing it improves problem-solving and code quality.
Topics to master:
- Arrays, Strings, Linked Lists
- Stacks, Queues
- Trees, Graphs, Tries
- Sorting Algorithms: Quick, Merge, Insertion, Selection
- Searching: Binary search, Linear search
- Hashing
- Recursion and Dynamic Programming
🎯 Practice on Codeforces, LeetCode, and GeeksforGeeks.
e)Build a C++ Project Using Qt
Creating a C++ project with Qt is the perfect fusion of high-performance backend logic and a powerful cross-platform GUI framework. Qt empowers developers to bring their C++ code to life with intuitive interfaces, event-driven programming, and modular architecture.
In this approach, core functionality is implemented using the robustness of C++, while Qt handles the presentation layer through its flexible widgets, signals and slots mechanism, and UI design tools. Whether it’s a data visualization dashboard, a custom tool, or a real-time system monitor, Qt makes it easy to integrate rich user experiences with efficient native code.
Building a project with Qt not only sharpens object-oriented programming skills but also teaches practical concepts like multi-threading, event handling, resource management, and cross-platform deployment—all in a unified development environment.
Qt is widely used in embedded GUIs (especially for infotainment, industrial devices, dashboards).
Steps:
- Install Qt Creator and set up a CMake-based project
- Learn Qt Widgets or QML for UI
- Connect buttons, sliders, and sensors using
signals
andslots
- Use multithreading with
QThread
orQtConcurrent
- Integrate serial communication with
QSerialPort
(to talk to microcontrollers) - Apply OOP design patterns (Observer, Singleton, etc.)
Project Ideas:
- Sensor Dashboard using Serial + Plot
- Embedded Device Simulator (LEDs, buttons)
- Audio Player UI (with QMediaPlayer)
- Home automation GUI to control ESP32/Arduino via serial
🛠 Bonus: Try to cross-compile the Qt app for ARM/Linux target (e.g., Raspberry Pi or BeagleBone).on bare-metal safe subsets.
2.3 Assembly Language (Bonus)
Assembly Language is the closest human-readable form of programming that directly interacts with a system’s hardware. It acts as a thin layer between machine code and high-level programming, providing fine-grained control over a processor’s operations.
Each instruction in Assembly corresponds to a single machine-level command, allowing developers to manipulate registers, memory addresses, I/O ports, and control flow with exact precision. This low-level access makes Assembly indispensable for performance-critical, time-sensitive, and resource-constrained environments such as embedded systems, operating systems, and device drivers.
Learning Assembly Language offers deep insights into CPU architecture, instruction sets, and how software translates into actual machine operations. It is not just a programming tool—it’s a window into the very core of computing.
When ultra-precise control is needed:
- Learn basic ARM Cortex-M Assembly (or AVR)
- Understand registers, stack frames
- Learn how to optimize critical sections
2.4 Python for Embedded Automation and Tools
Python has become an indispensable tool in the embedded systems world, offering a high-level language that enhances productivity while maintaining the low-level precision required for embedded automation. By leveraging Python in embedded environments, developers can rapidly prototype, automate processes, and streamline toolchains without sacrificing performance.
Python excels in creating custom automation scripts, interacting with hardware interfaces, and building tooling that simplifies development workflows. Whether it’s controlling GPIO pins, interfacing with sensors, or automating testing procedures, Python brings simplicity and flexibility to embedded systems, making tasks like data logging, hardware testing, and system monitoring much more efficient.
With libraries such as RPi.GPIO, PySerial, and PyTest, Python enables seamless integration between embedded platforms and the tools used for development, testing, and debugging. It’s the perfect language for rapid development and automation, bridging the gap between embedded hardware and sophisticated software control.
Python isn’t usually used directly on MCUs but is great for:
- Writing test scripts for embedded hardware
- Serial communication (
pyserial
) - Parsing logs, files, and test reports
- GUI with
Tkinter
orPyQt
- Data plotting with
matplotlib
Embedded Use Cases:
- Automate firmware flashing over USB/UART
- Data logger scripts
- Backend control panel for IoT devices
- Unit test automation
2.5 Shell Scripting (Bash) for Embedded Linux
Shell scripting, particularly with Bash, is a powerful and flexible tool for managing and automating tasks in Embedded Linux environments. It allows developers to streamline system administration, automate device configurations, and control the boot process—all through simple, concise commands.
In the realm of embedded systems, Bash scripts serve as the bridge between hardware and software, facilitating tasks like system startup configuration, file manipulation, hardware diagnostics, and process management. The efficiency of Bash lies in its ability to manipulate files, manage system resources, and interact with the Linux kernel—all without needing to write complex C code.
A must-have for embedded Linux developers:
- Basics:
if
,for
,while
, variables, functions - File operations, logging, redirections
- Using
grep
,awk
,sed
,cut
- Automate build, flash, and deploy pipelines
- Write scripts to configure GPIO, I2C, or SPI on Linux boards
Used heavily in Yocto, Buildroot, init scripts, and service management.
Step 3: Systems Programming and Embedded Driver Development with ARM Cortex M3/M4
Microcontroller & Peripheral Programming
Basic Embeddded Topic
- What is a Microcontroller (MCU)?
- Common architectures: AVR, ARM Cortex-M, RISC-V
- MCU vs MPU vs SoC
- Clock system
- Counter
- Interrupts
- Polling
- Timer
- System Tick Timer (SysTick)
- Watchdog Timer
- GPIO (General Purpose Input/Output)
- ADC (Analog to Digital Converter)
- DAC (Digital to Analog Converter)
- PWM (Pulse Width Modulation)
- Low Power Modes
- DMA (Direct Memory Access)
- Clock Management
- EEPROM / Flash Memory Access
- Memory-Mapped Registers
- Linker Script Awareness
Note : To gain a deeper understanding of Systems Programming on ARM Cortex-M3/M4 MPU, it is highly recommended to engage in hands-on practical exercises
Communication Protocol
- I2C
- SPI
- UART / USART
- I2S
- TC/IP
- UDP
- Ethernet
- CAN
- LIN
- BT / WIFI / USB
Note : For an in-depth understanding, consider mastering microcontroller development with a focus on embedded driver development, including I2C, GPIO, USART, timers, PWM, CAN, RTC, STM32-LTDC, LCD-TFT, and LVGL.
Popular MCUs to practice:
- Atmega328p (Arduino Uno)
- STM32F103, STM32F4 series
- ESP32 (for Wi-Fi + BLE)
- NXP, Renesas, TI MCUs
Step 4: Driver Development on the Operating System (OS) and Build System
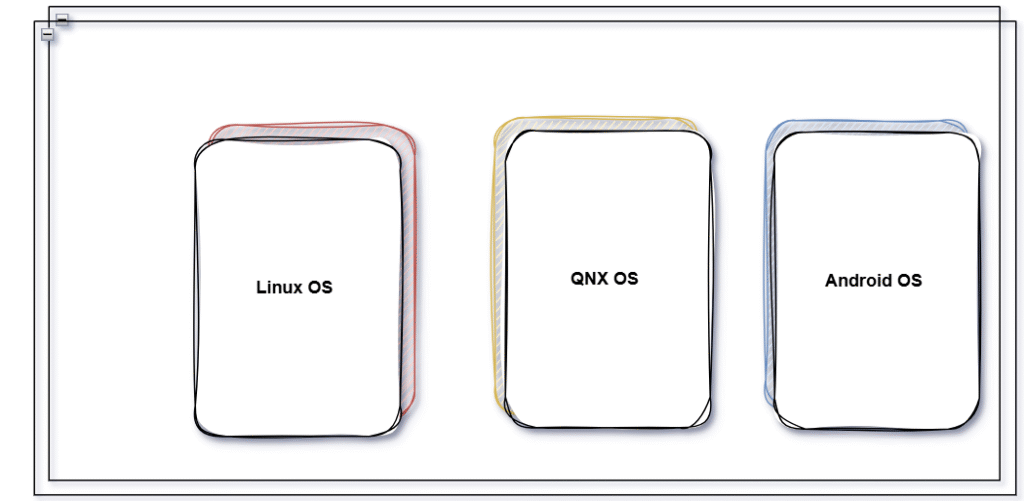
Linux System Programming , Kernel Programming and Drivers devlopment
- Linux Kernel Architecture
- Kernel Modules
- Device Drivers Overview
- Character Device Drivers
- Block Device Drivers
- Interrupt Handling in Linux
- GPIO Driver Development
- I2C Driver Development
- SPI Driver Development
- UART Driver Development
- PWM Driver Development
- DMA in Linux Drivers
- Power Management in Linux Drivers
- File Operations in Device Drivers
- Linux Kernel Debugging (using
dmesg
,gdb
, etc.) - System Calls & User Space Communication
- PCIe Device Drivers
- USB Device Drivers
- Networking Drivers
- Device Tree & DTS files
RTOS (Real-Time Operating System) [FreeRTOS or QNX]
- RTOS Architecture & Basics
- Task Scheduling in RTOS
- Interrupt Handling in RTOS
- RTOS Memory Management
- Semaphore, Mutex, and Event Flags
- RTOS Timers
- RTOS for Embedded Systems
- Bare-Metal vs RTOS Applications
- RTOS Device Drivers
- RTOS-Specific I/O Driver Development
- RTOS with FreeRTOS / embOS / Micrium
- Inter-Process Communication in RTOS
- RTOS Power Management
- RTOS Porting to Custom Hardware
- RTOS and Network Stack Integration (e.g., lwIP)
QNX Interview Question Link : Master QNX OS Interview Questions
Bootloaders
- Boot Process Overview
- U-Boot Basics
- Customizing U-Boot for Embedded Systems
- Bootloader Initialization
- Bootloader Device Drivers
- Bootloader and Kernel Image Management
- Bootloader for Flash Memory
- Bootloader Debugging and Troubleshooting
Compiler & Toolchain (GCC)
- GCC Command-line Options
- GCC Compilation Stages (
Preprocessing
,Compilation
,Assembly
,Linking
) - Cross Compilation
- Linking and Object Files (
.o
,.a
,.so
) - Optimization Flags
- Debug Flags & Symbols (
-g
,-O0
to-O3
) - Linker Scripts (Basics)
- Toolchain Components:
as
,ld
,objdump
,nm
,readelf
Build System
- Makefile Basics
- Makefile Variables, Targets, and Rules
- Makefile for Embedded Projects
- CMake Basics
- Writing
CMakeLists.txt
- CMake vs Make
- Cross-compilation with CMake
Bash Scripting
- Shell Scripting Basics
- Conditional Statements and Loops
- Functions in Shell
- Script for Build Automation
- Script for Device Flashing
- Logging and Error Handling in Scripts
Docker
- Docker Basics & Architecture
- Creating Dockerfiles
- Docker Images vs Containers
- Building and Running Embedded Dev Environments
- Docker Volumes and Networking
- Using Docker for Cross-compilation
- Docker Compose (Optional)
Yocto Project
- Yocto Basics & Directory Structure
- BitBake and Recipes
- Layers, Metadata, and Machine Config
- Writing a Simple BitBake Recipe
- Adding a Custom Layer
- Building a Minimal Linux Image
- Adding Kernel Modules or Drivers
- Deploying to Hardware
- Yocto with U-Boot & Device Tree
- Debugging Yocto Builds
Step 5: Software Design, Debugging, Testing & SDLC
Design & Architecture 🧠
- UML Diagrams (Use Case, Class, Sequence, Activity)
- UML State Machine Diagrams
- Finite State Machines (FSM)
- Software Architecture Patterns (Layered, Modular, etc.)
- Real-Time System Design Concepts
Version Control 🧾
- Git Basics (
clone
,commit
,push
,pull
) - Branching & Merging
- Git Workflow (Feature Branch, Git Flow)
- Conflict Resolution
- Git Rebase vs Merge
- Git Tags and Releases
Debugging Tools 🐞
- SWD (Serial Wire Debug)
- JTAG (Joint Test Action Group)
- GDB Basics and Commands
- Remote Debugging with GDB
- Breakpoints, Watchpoints, Backtrace
- Disassembly and Register Inspection
- Debugging on Bare-Metal & RTOS
SDLC (Software Development Life Cycle) 🔄
- Requirements Analysis
- Design Phase
- Implementation Phase
- Testing & Integration
- Maintenance Phase
- Agile Model
- Waterfall Model (for comparison)
Testing & Quality ✅
- Test Driven Development (TDD)
- Unit Testing Frameworks (Google Test, Unity)
- Integration Testing Basics
- System Testing
- Static Code Analysis Tools (Cppcheck, Clang-Tidy, PC-lint)
- MISRA-C / MISRA-C++ Guidelines
- Code Coverage Tools
- Continuous Integration Basics (Jenkins, GitHub Actions)
Test Equipment Usage 🔬
- Using a Multimeter (Voltage, Current, Continuity)
- Using a Logic Analyzer (Protocol Decoding: UART, SPI, I2C)
- Using an Oscilloscope (Waveform Analysis, Triggering, Noise Detection)
- Using Power Supply and Lab Tools Safely
Board Bring-up Skills 🧰
- Power-On Checks and Voltage Rails
- Clock Signal Verification
- Reset Circuit Validation
- Boot Logs & Serial Debugging
- Flashing Bootloaders and Firmware
- Verifying Peripheral Functionality
- Connectivity Tests (USB, Ethernet, CAN)
- Interface Testing (UART, I2C, SPI, GPIO)
Step 6: Embedded System Job Roles
Core Embedded Development 🔧
- Embedded Software Engineer
- Firmware Engineer
- Embedded Systems Developer
- Bare-metal Embedded Developer
- RTOS Developer
Application & Middleware 💻
- Embedded C++ Application Developer
- Middleware Software Engineer
- Qt Application Developer (for GUI on embedded devices)
- HMI Developer (Human Machine Interface)
Driver and OS Layer ⚙️
- Linux Device Driver Developer
- Kernel Developer (Linux/RTOS)
- Board Support Package (BSP) Engineer
- Embedded Linux Engineer
- RTOS Kernel/Porting Engineer
Communication & Protocols 📶
- Protocol Stack Developer (CAN, UDS, TCP/IP, etc.)
- IoT Firmware Developer
- Connectivity Engineer (Bluetooth, Zigbee, Wi-Fi, LoRa)
- Wireless Communication Engineer
Testing and QA 🔬
- Embedded Test Engineer
- Validation & Verification Engineer
- Hardware-in-the-Loop (HIL) Test Engineer
- Automation Test Engineer (Python, Robot Framework)
- Software Quality Engineer (Static/Dynamic Analysis)
Debug & Diagnostics 🔍
- Embedded Debugging Engineer
- Field Application Engineer (FAE)
- Diagnostics Software Engineer (UDS, ISO 14229)
Hardware-Software Co-design 📡
- Embedded Hardware Engineer
- FPGA Developer (Verilog/VHDL)
- SoC Integration Engineer
- PCB Bring-up and Debug Engineer
AI/ML and Embedded Intelligence 🧠
- Embedded AI/ML Developer (TinyML)
- Edge Computing Engineer
- Vision-based Embedded Developer (OpenCV, TensorFlow Lite)
Build, Release, and Automation 📦
- Yocto Build Engineer
- DevOps for Embedded Systems
- CI/CD Engineer (for Embedded Projects)
- Toolchain & Compiler Specialist
Project Management & Process 🧾
- Embedded Project Manager
- Scrum Master (for Embedded Teams)
- ASPICE/AUTOSAR Compliance Engineer
Special thanks to @mr-raj for contributing to this article on Embedded Prep
1. What is embedded software?
Embedded software is a specialized type of software designed to run on hardware devices like microcontrollers, sensors, or boards such as Arduino, STM32, or Raspberry Pi. It controls specific functions within a larger system, often in real-time.
2. Is this roadmap suitable for complete beginners?
Yes! This roadmap is designed for absolute beginners with no prior experience in embedded systems. Whether you’re a student or a career switcher, you’ll find easy-to-follow steps, project ideas, and learning paths.
3.Do I need to know C or C++ to get started?
Knowing C is highly recommended, as it’s the foundation of embedded programming. However, our roadmap starts from scratch and will guide you to learn C and eventually C++ at a comfortable pace.
4.What hardware do I need as a beginner?
You can start with basic, affordable boards like:
Arduino Uno
ESP32 or ESP8266 (for IoT projects)
STM32 (for advanced learning)
These boards are beginner-friendly and widely supported by tutorials.
5.Do I need electronics knowledge to start embedded programming?
Only basic electronics knowledge is needed. The roadmap includes beginner-friendly explanations of resistors, capacitors, GPIOs, sensors, and circuits with diagrams and examples.
6.Can I follow this roadmap while working or studying full-time?
Absolutely! The guide is broken down into Step wise milestones, so you can learn at your own pace — even if you have just a few hours per week.
7.What tools and software are covered in the roadmap?
You’ll learn how to use:
Arduino IDE
PlatformIO
STM32CubeIDE
QEMU (for emulation)
Git & GitHub
Linux terminal basics
GCC compiler and Makefiles
8.Does the roadmap include real-world projects?
Yes! You’ll work on practical projects like:
LED blinking
Temperature & humidity monitor
IoT gas alert system
Audio player with amplifier
Real-time sensor dashboard with Python and web interface
9.Is Linux knowledge required?
Basic Linux knowledge is helpful but not mandatory. The roadmap includes a beginner section to get you comfortable with Linux commands, scripting, and embedded Linux fundamentals.
10.What’s the goal of this roadmap?
The roadmap aims to help you:
✅ Build a strong foundation in embedded systems
✅ Learn to work with real hardware
✅ Understand drivers, RTOS, and protocols
✅ Create portfolio-worthy projects
✅ Prepare for embedded job interviews
11.Where can I find the full roadmap?
The complete guide is available on our website/blog: Embedded Prep Master – Make sure to bookmark it and follow us for updates!
You can also Visit other tutorials of Embedded Prep
- What is eMMC (Embedded MultiMediaCard) memory ?
- Top 30+ I2C Interview Questions
- Bit Manipulation Interview Questions
- Structure and Union in c
- Little Endian vs. Big Endian: A Complete Guide
- Merge sort algorithm
Special thanks to @mr-raj for contributing to this article on Embedded Prep
Leave a Reply